React has a different approach to data flow and manipulation than other frameworks, and that’s why it can be difficult in the beginning to understand concepts like props, state and others.
React Props Definition
Props is a special keyword in React that stands for properties and is used for passing data from one component to another. Data with props are passed in a unidirectional flow from parent to child.
We’re going to focus on React’s Props feature and how to use it. Props is a special keyword in React that stands for properties, and it’s used for passing data from one component to another.
To understand how props work, first, you need to have a general understanding of the concept of React components. We’ll cover that and more in this article.
What Is React Props?
React is a component-based library that divides the UI into little reusable pieces. In some cases, those components need to communicate or send data to each other, and the way to pass data between components is by using props.
As I shared, props
is a keyword in React that passes data from one component to another. But the important part here is that data with props are being passed in a unidirectional flow. This means it’s passed one way from parent to child.
Props data is read-only, which means that data coming from the parent shouldn’t be changed by child components.
Now, let’s see how to use props
with an example.
How to Use Props in React With Example
I will be explaining how to use props
step-by-step. There are three steps to using React props
:
- Define an attribute and its value (data).
- Pass it to the child component(s) by using
props
. - Render the
props
data.
In this example, we have a ParentComponent
including another ChildComponent
:
class ParentComponent extends Component {
render() {
return (
<h1>
I'm the parent component.
<ChildComponent />
</h1>
);
}
}
And this is our ChildComponent
:
const ChildComponent = () => {
return <p>I'm the 1st child!</p>;
};
The problem here is that when we call the ChildComponent
multiple times, it renders the same string again and again:
class ParentComponent extends Component {
render() {
return (
<h1>
I'm the parent component.
<ChildComponent />
<ChildComponent />
<ChildComponent />
</h1>
);
}
}
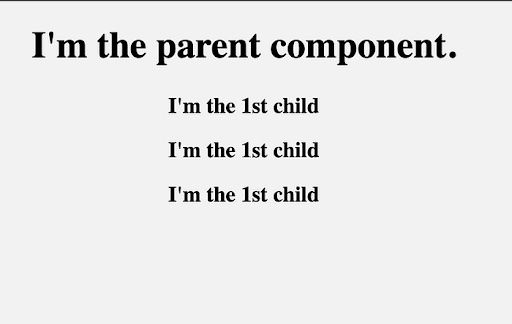
But what we like to do here is to get dynamic outputs, because each child component may have different data. Let’s see how we can solve this issue by using props
.
1. Defining Attribute & Data
We already know that we can assign attributes and values to HTML tags:
<a href="www.google.com">Click here to visit Google</a>;
Likewise, we can do the same for React components. We can define our own attributes and assign values with interpolation { }
:
<ChildComponent someAttribute={value} anotherAttribute={value}/>
Here, I’m declaring a text
attribute to the ChildComponent
and then assign a string value: “I’m the 1st child”
.
<ChildComponent text={“I’m the 1st child”} />
Now, the ChildComponent
has a property and a value. Next, we need to pass it via props
.
2. Pass Data Using Props
Let’s take the “I’m the 1st child!” string and pass it by using props
.
Passing props
is very simple. Just as we pass arguments to a function, we pass props
into a React component and props
brings all the necessary data. Arguments passed to a function:
const addition = (firstNum, secondNum) => {
return firstNum + secondNum;
};
Arguments passed to a React component:
const ChildComponent = (props) => {
return <p>I'm the 1st child!</p>;
};
3. Rendering Props Data
We’ve created an attribute and its value, then we passed it through props
, but we still can’t see it because we haven’t rendered it yet.
A prop
is an object. In the final step, we will render the props object by using string interpolation: {props}
.
But first, log props
to console and see what it shows:
console.log(props);

As you can see, props
returns an object. In JavaScript, we can access object elements with dot notation. So, let’s render our text property with an interpolation:
const ChildComponent = (props) => {
return <p>{props.text}</p>;
};
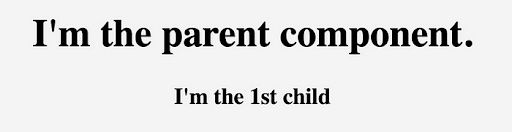
And that’s it. We’ve rendered the data coming from the parent component. Before closing, let’s do the same for other child components:
class ParentComponent extends Component {
render() {
return (
<h1>
I'm the parent component.
<ChildComponent text={"I'm the 1st child"} />
<ChildComponent text={"I'm the 2nd child"} />
<ChildComponent text={"I'm the 3rd child"} />
</h1>
);
}
}
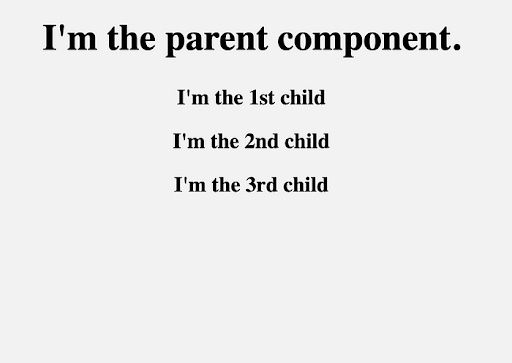
As we can see, each ChildComponent
renders its own prop data. This is how you can use props
for passing data and converting static components into dynamic ones.
Understanding React Props
To recap:
- Props stand for properties and is a special keyword in React.
- Props are being passed to components like function arguments.
- Props can only be passed to components in one way (parent to child).
- Props data is immutable (read-only).
Understanding React’s approach to data manipulation takes time. I hope my post helps you to become better at React.